# 범위
- 프로토타입 객체의 정의
- 프로토타입 객체 생성 방법1 : new Object()
- 프로토타입 객체 생성 방법2 : 리터럴 표기법
- 프로토타입 객체 생성 방법3 : 생성자 메소드
# 노트 :
● 프로토타입 객체의 정의 , 프로토타입 객체 생성 방법1 : new Object()
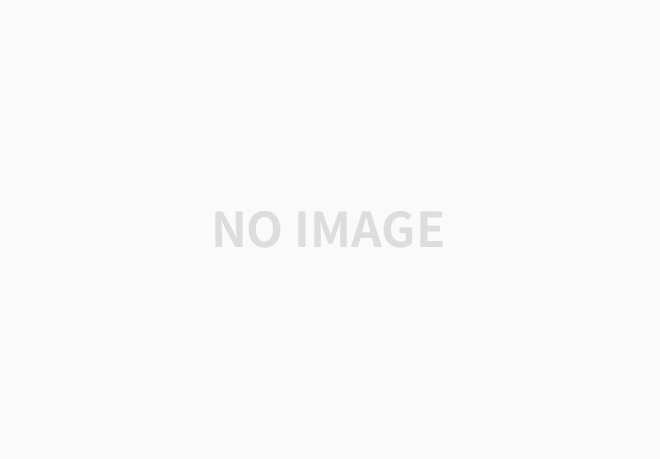
● 프로토타입 객체 생성 방법2 : 리터럴 표기법
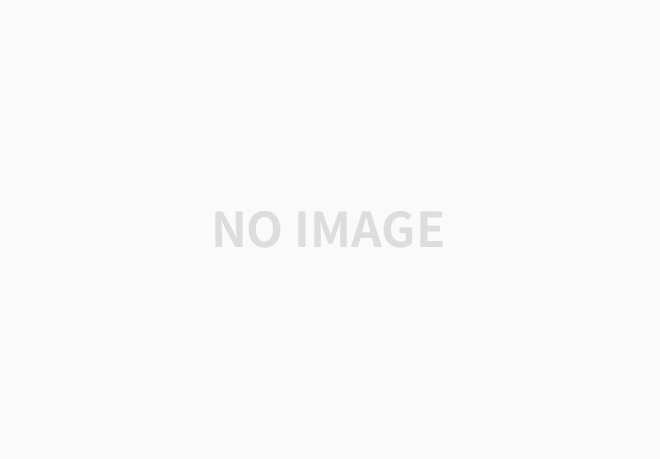
● 프로토타입 객체 생성 방법3 : 생성자 메소드
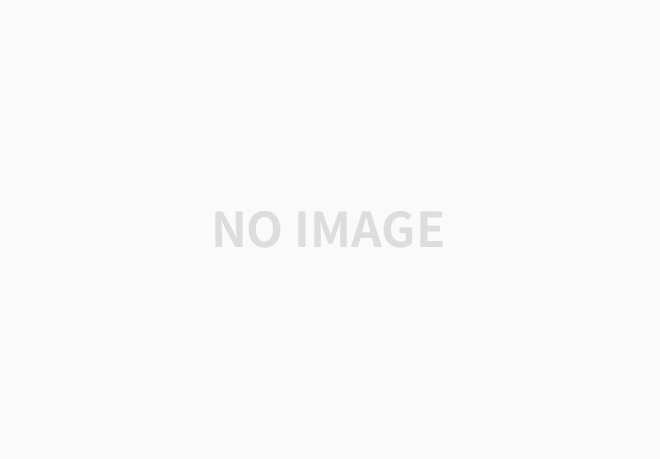
● 연습_방법1,2,3으로 account 객체 만들기




○ 요약 :
※ 이건 요약을 하는 것보다, 코드로 기억을 떠올리는 것이 좋을 것 같다.
● 프로토타입 생성 방법1_ new Object() :
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
|
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Account객체 만들기</title>
<script>
let account = new Object();
account.owner = "황기태";
account.code = "111";
account.balance = 35000;
account.inquiry = function(){
return this.balance;
}
account.deposit = function(cash){
this.balance +=cash;
}
account.withdraw = function(cash){
this.balance -=cash;
}
</script>
</head>
<body>
<script>
document.write("account : "
+account["owner"]+","
+account["code"]+","
+account["balance"]
+"<br>");
account.deposit(10000);
document.write(
"10000원 저금 후 잔액은 "
+account["balance"]
+"<br>"
);
account.withdraw(5000);
document.write(
"5000원 인출 후 잔액은 "
+account["balance"]
+"<br>"
);
</script>
</body>
</html>
|
cs |
● 프로토타입 생성 방법2_ 리터럴 표기법 :
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
|
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Account객체 만들기</title>
<script>
let account = {
owner : "황기태",
code : "111",
balance : 35000,
inquiry : function()
{
return this.balance;
},
deposit : function(cash)
{
this.balance +=cash;
},
withdraw : function(cash)
{
this.balance -=cash;
}
};
</script>
</head>
<body>
<script>
document.write(
"acccount : "
+account.owner+", "
+account.code+", "
+account.balance
+"<br>"
)
account.deposit(10000);
document.write(
"10000원 저금 후 잔액은 "
+account.inquiry()
+"<br>"
)
account.withdraw(5000);
document.write(
"5000원 저금 후 잔액은 "
+account.inquiry()
+"<br>"
)
</script>
</body>
</html>
|
cs |
● 프로토타입 생성 방법3_ 생성자 메소드 :
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
|
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Account객체 만들기</title>
<script>
function Account(name, code, balance) {
this.owner = name;
this.code = code;
this.balance = balance;
this.inquiry = function () {
return this.balance;
};
this.deposit = function (cash) {
this.balance += cash;
};
this.withdraw = function (cash) {
this.balance -= cash;
};
}
</script>
</head>
<body>
<script>
let account1 = new Account("황키태", "111",35000);
document.write("account : "
+ account1.owner + ","
+ account1.code + ","
+ account1.balance+"<br>"
);
account1.deposit(10000);
document.write("10000원 저금 후 잔액은 "
+account1.inquiry()+"<br>"
);
account1.withdraw(5000);
document.write("5000원 인출 후 잔액은 "
+account1.inquiry()+"<br>"
);
</script>
</body>
</html>
|
cs |
'웹프로그래밍 > 웹프로그래밍수업' 카테고리의 다른 글
(34)명품 웹프로그래밍_7장_실습문제_주요문제만(4번,7번,8번,10번) (4) | 2024.10.20 |
---|---|
(32)명품 웹프로그래밍_7장_코어객체와 객체 생성_1 (2) | 2024.10.20 |
(30)명품 웹프로그래밍_6장_자바스크립트언어_2 (0) | 2024.10.13 |
(30)명품 웹프로그래밍_6장_자바스크립트언어_1 (0) | 2024.10.12 |
(29)명품 웹프로그래밍_5장_Open_Challenge (5) | 2024.10.12 |